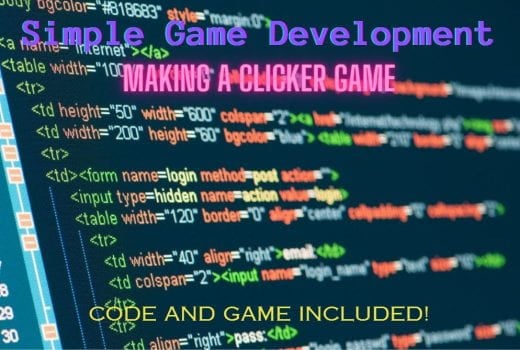
By: Nathaniel Cole
Do you ever wonder “How do I make a game with code?” This article is for you if you do (or even if you don’t!). I will show you how to write an HTML clicker game, and even make it look good in part 2!
Subsection 1; What is HTML
If you already know the syntax of HTML, you can skip to subsection 2, otherwise this will help you program even more games and start your programming journey! HTML consists of tags, which are <code><tag name></code> some tags have special attributes that can be used by putting <code><tag name attributename=attribute><code/> HTML is nested, which means you put tags in tags. If you want a more complicated overview of HTML please go to https://www.w3schools.com/html/
Some facts about HTML
- HTML stands for Hypertext Markup Language
- HTML IS NOT A PROGRAMMING LANGUAGE
- HTML describes the structure of a webpage
- The labels in HTML tags describe what the tag does
Important Tags
The tags we’ll be using are <code><h1></code>, <code><span></code>, and <code><button></code>. These tags are important because it’s all the tags we use. The h1 tag gives big text, the span element cannot create a new line when put in, this is useful for changing its data. The button tag is useful because it runs JavaScript code every time it’s clicked.
With these new tags, we can make a simple clicker game.
Creating HTML (the format)
The way I will display my HTML is by using it embedded, if you want to try your own at the bottom of this article there is a simple HTML viewer. All HTML has to have a <head> and <body> tag. Your HTML will not need a body tag, because the HTML you will writing is in the body of the webpage, so start with what you would like to have inside the body. If you’re running a web server, you’ll need the full <html> format.
JavaScript
Javascript is an important part of writing games, create dynamic HTML that can do anything to your will. With JavaScript, you can easily create clicker games and even more advanced games where you click and particles appear.
I’ll provide a simple understanding of JavaScript, but if you have any questions about functions you should look them up on MDN docs. Technically speaking, it’s for Mozilla Firefox, but for JavaScript functions, it all works as long as you have javascript ES7.
The functions and keywords we’ll use in our code are the following:
- var | keyword | creates a variable of your name (var varname; or var varname = varvalue)
- function | keyword? | creates a function of your name. Use braces ({}) to put the function’s code in it.
- document | variable | document is the website
- getElementById | function | part of document | func(idname) -> element
The code (writing)
Now we are ready to write the code. First, we need a way to see how many times we clicked, otherwise we may as well have a button that does nothing! We’ll put an <h1> tag. This will create big text. In the <h1> we’ll have a <span> which is inline (meaning it stays on the same line) Let’s put “clicks” into the <h1> and make the spans ID “score.” Span has a default value you can put inside of the tag which shows before a javascript update. This means at the top of your webpage put “<h1>Clicks: <span id=”score”>0</span></h1>”
Now that the display is finished we can create the button you click. Buttons are created with the <button> tag. This will put something clickable that runs JavaScript on click. Lets use the function we’ll define as “clicked()” <button onclick=”clicked()”>Click me!</button>
Now we need to write the javascript, which is usually the second hardest, the hardest being figuring out what to make! We want our JavaScript to do is in our “clicked()” function, it needs to increase the clicked by one and and update our span.
Lets create a variable telling use how many times you’ve pressed that button. To initialize the variable you need to use the “var” keyword. Now we make the clicked function for when the button is pressed. Increase the clicks by one then update the span. You need to get the element first, and you can do this by using document.getElementById. This will give you the element where you can change it’s text with the innerHTML property. Just set that to your clicks.
Now we’ve finished with our game! You can test your game out at the bottom if this page using my html renderer.
Here is the finished code!
<code>
<h1>Clicks: <span id=”score”>0</span></h1>
<button onclick=”clicked()”>Click me!</button>
<script>
var clicks = 0;
function clicked() {
clicks += 1;
document.getElementById(“score”).innerHTML = clicks;
}
</script>
</code>
<code><h1>Clicks: <span id=”score”>0</span></h1>
<button onclick=”clicked()”>Click me!</button>
<script>
var clicks = 0;
function clicked() {
clicks += 1;
document.getElementById(“score”).innerHTML = clicks;
}
</script></code>
Now for how you can test your own!
<textarea id=”code” placeholder=”type your code!”></textarea>
<p id=”rendered”>No code written</p>
<script>
setInterval(() => {
document.getElementById(“rendered”).innerHTML = document.getElementById(“code”).value;
}), 200);
</script>